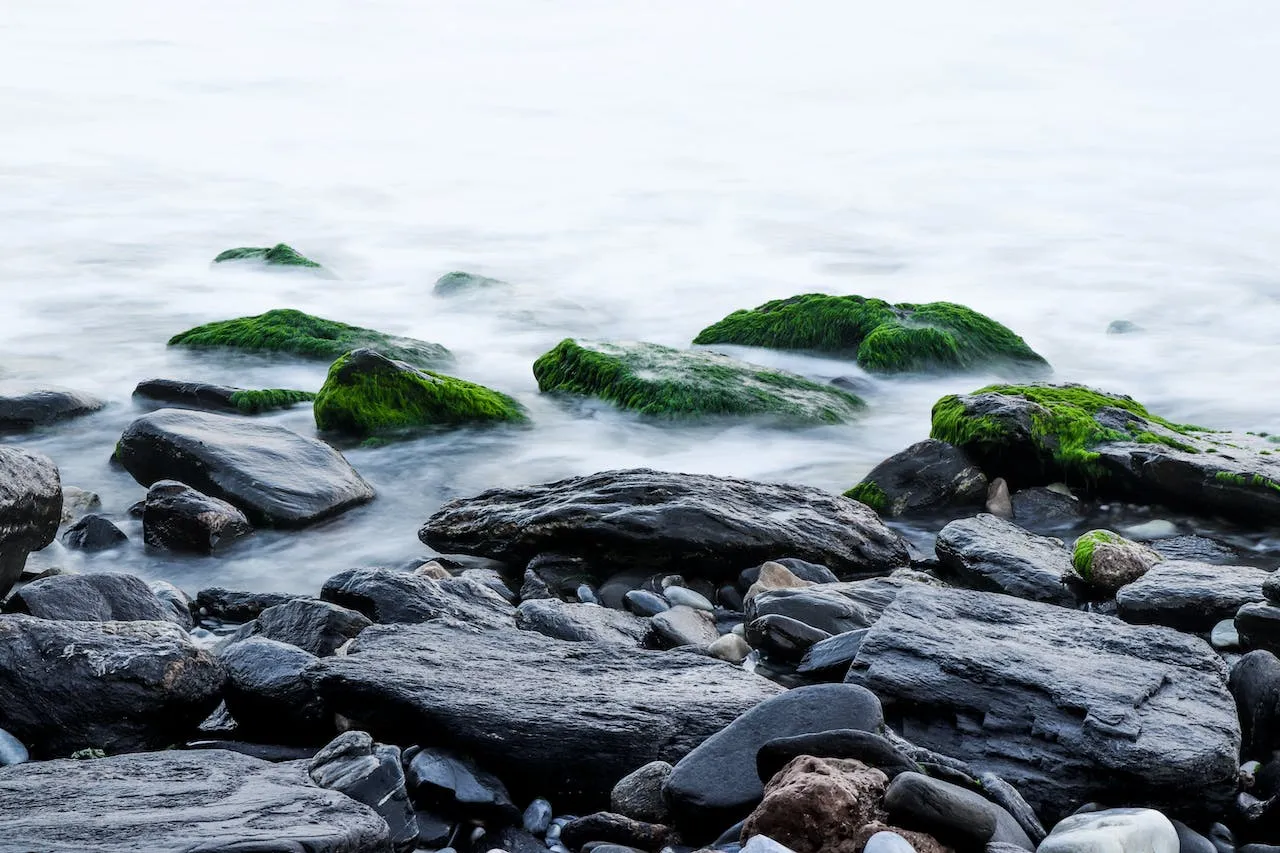
A java.lang.NullPointerException
is a common error encountered by Java developers. It indicates that the code is attempting to access or modify an object reference that’s not initialized, resulting in a “null” value.
What are Null Pointer Exceptions ?
What is the definition of Null Pointer Exceptions (java.lang.NullPointerException) and what are the factors that lead to their occurrence? Let’s discuss on this
In Java, there are two main categories of variables:
- Primitives: These variables hold data directly. Consequently, to manipulate the data stored in a primitives variable, you can directly modify the variable itself. Primitives, albeit basic, are typically named with a lowercase letter as per convention. For instance, variables of types like int or char are considered primitives.
- References: In contrast, these variables store the memory address of an Object, meaning they refer to an Object. Therefore, to manipulate the Object that a reference variable points to, you need to dereference it. Dereferencing often involves using the “.” operator to access a method or field, or using “[” to index an array. Reference types, though more complex, are commonly denoted with a type that starts with an uppercase letter. For example, variables of type Object are considered references.
Take into account the following code snippet in which you declare an uninitialized variable of primitives type int:
int h;
int j = h + h;
The program will encounter a crash when these two lines are executed because the variable “h” has not been assigned a value. As a result, errors will occur when we try to use the value of “h” to define “j”. It is crucial to initialize all Primitives variables with a valid value before performing any operations on them.
Additional Details
Now, let’s delve into an intriguing aspect. Reference variables have the ability to be assigned a special value called ‘null,’ essentially meaning ‘I am not referring to anything.’ Additionally, you can obtain a null value in a reference variable through explicit assignment or when a reference variable is uninitialized, and the compiler fails to identify it (Java automatically sets such variables to null).
If a reference variable is explicitly set to null by you or automatically by Java, and you attempt to access it, you will encounter a java.lang.nullpointerexception. Consequently, the java.lang.nullpointerexception(NPE) commonly arises when you declare a variable but fail to create an object and assign it to the variable before attempting to access the variable’s contents. In other words, you have a reference to something that does not actually exist.
Consider following code for explanation
Integer fun;
fun = new Integer(30);
Initially, in the first line of code, a variable named ‘fun’ is declared, albeit without containing a reference value yet. Because no specific value is assigned to it, Java automatically sets it to null.
Subsequently, moving on to the second line, the ‘new’ keyword is used to instantiate an object of the Integer type, and the reference variable ‘fun’ is then assigned to this newly created Integer object.
However, if you attempt to access the contents of ‘fun’ before creating the object, a java.lang.nullpointerexception will occur. In simpler cases, the compiler will detect this issue and prompt you with a message stating that ‘fun may not have been initialized.’ Nevertheless, there might be situations where you write code that does not directly create the object, potentially leading to issues.
Understanding NullPointerException
What Causes It?
This error often occurs when a method or object is called on an object that hasn’t been instantiated. Consequently, it can happen due to various reasons, such as uninitialized variables, incorrect object references, or when a method returns null.
Learn how to fix javascript error in the article “Avoid “javascript:void(0)” for Empty Links – Best Practices for SEO and User Experience“.
List of situations that cause a NullPointerException
to occur
Here are all the scenarios explicitly mentioned in the Java Language Specification in which a java.lang.nullpointerexception can occur:
- Accessing (i.e., getting or setting) an instance field of a null reference. However, note that this doesn’t apply to static fields!
- Calling an instance method of a null reference. This exclusion doesn’t apply to static methods!
- Throwing null explicitly, such as with ‘throw null;’
- Accessing elements of a null array.
- Synchronizing on null, denoted as synchronized (someNullReference) { … }
- Any integer or floating-point operator can throw a NullPointerException if one of its operands is a boxed null reference.
- Unboxing conversion throws a NullPointerException if the boxed value is null.
- Calling super on a null reference throws a NullPointerException. Specifically, this refers to qualified superclass constructor invocations.
class Outer {
class Inner {}
}
class ChildOfInner extends Outer.Inner {
ChildOfInner(Outer o) {
o.super(); // if o is null, NPE gets thrown
}
}
However, it’s important to note that these situations are directly stated in the Java Language Specification.
Here are additional cases where a NullPointerException can occur, as mentioned by the Java Language Specification:
- Using a for-each loop (for element : iterable) to iterate through a null collection or array.
- The switch statement (whether used as an expression or statement) can throw a NullPointerException when the switch expression (foo) is null.
- Invoking foo.new SomeInnerClass() throws a NullPointerException when foo is null.
- Method references in the form of name1::name2 or primaryExpression::name can throw a NullPointerException when evaluated and either name1 or primaryExpression evaluates to null.
It’s important to note that according to the JLS, someInstance.someStaticMethod() does not throw a NullPointerException because someStaticMethod is static. However, using the method reference syntax someInstance::someStaticMethod can still throw a NullPointerException if someInstance is null.
Impact of the Exception
NullPointerExceptions, although seemingly small, can critically disrupt a Java program’s flow. Consequently, if left unaddressed, they can result in program crashes or unforeseen behavior.
Fixing the Issue
Identifying the Problem:
Problem Code:
String name = null;
int length = name.length(); // This line will throw a NullPointerException
Solution: Null Check
String name = null;
if (name != null) {
int length = name.length(); // Executes only if 'name' is not null
} else {
// Handle the situation where 'name' is null
}
Defensive Coding:
Always check for null values before accessing object properties or invoking methods on an object. Employing if-else conditions or try-catch blocks can prevent this error. Not only can checking for null values before accessing object properties enhance code reliability, but also employing if-else conditions or try-catch blocks is crucial to prevent potential errors. Additionally, by incorporating if-else conditions or try-catch blocks, null value-related issues can be mitigated effectively. Whether you’re using if-else conditions or try-catch blocks, preventing null value errors is essential for robust code. On the one hand, checking for null values beforehand is crucial; on the other hand, using if-else conditions or try-catch blocks provides a safety net against potential errors. Furthermore, both checking for null values and utilizing if-else conditions or try-catch blocks are vital strategies to ensure code stability and prevent unexpected errors.
Null Checks:
Validate user inputs, method returns, and object references. Additionally, implement defensive programming by verifying data before operations. This ensures robustness against errors.
Problem Code:
public void printLength(String text) {
int length = text.length(); // This line might throw a NullPointerException if 'text' is null
System.out.println("Length: " + length);
}
Solution: Null Check
public void printLength(String text) {
if (text != null) {
int length = text.length(); // Executes only if 'text' is not null
System.out.println("Length: " + length);
} else {
System.out.println("Text is null");
}
}
Code Review:
Regularly reviewing code is essential to spot potential null reference issues. Consequently, enforcing strict guidelines on handling null references within development teams is crucial. By doing so, both identifying and mitigating such issues become more efficient.
Conclusion
A java.lang.NullPointerException is a prevalent error in Java programming. However, with careful coding practices and thorough null checks, it’s manageable and preventable. By implementing diligent handling of null values, occurrences of NullPointerExceptions can be significantly reduced, thereby enhancing the robustness of Java applications.